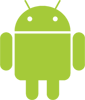
Basic Toast
Creating toasts requires only one line as shown below:Basically all you need to do is invoke the static method Toast.makeText(), which will create a Toast object. The first argument takes in the current activity's Context object, second argument is the message that you would like to display, and finally the third argument would be the length of time that you would like it to be displayed for. Currently, the third argument will only either accept Toast.LENGTH_SHORT or Toast.LENGTH_LONG and you will not be able specify your own set time. Once the Toast object is created, invoke Toast.show() with that Toast object. If you want to display Toast longer then just replace Toast.LENGTH_SHORT with Toast.LENGTH_LONG and you are done!
Display Toast Even Longer
Currently, I was only able to find one way to display Toasts longer than Toast.LENGTH_LONG. What I did was basically invoke show() on the same Toast object more than once consecutively. This was simply done by using a for loop and looping it as many times as you like. Depending on how many times you loop, it will display it for Toast.LENGTH_LONG times the number of times you loop.In the above code, I am looping 4 times which results to a Toast message displaying for 4×Toast.LENGTH_LONG amount of time.
Custom Toast Background
If you happen to not like the default background, you create your own custom background and display Toasts messages using that background. To do this, you will first need to create a 9-patch image that you want to use for your Toast background. One tool that you can use to create 9-patch images from an existing image is Nine-patch Generator. Once you have placed the 9-patch images to their corresponding folders in your Android project, you need to then create a separate layout for your Toast and set that 9-patch image as background value for your root layout. Like this:Creating a layout like the above gives you the flexibility on how you want to display your Toast. Once you have created your Toast message layout, next step is just a matter of writing few simple lines of code, shown below.
There is also another way customizing your Toast messages. This is done through Android's XML styling and more information can be found here in this link.
You can view my Android Toast example HERE.
Relevant files are:
src/com/choiboi/apiexamples/toast/ToastMainActivity.java
res/layout/activity_toast.xml
res/layout/activity_toast_custom_toast.xml
Happy Coding!